React Native testing works best with a layered approach: fast unit tests for business logic, focused component tests for UI behavior, and end‑to‑end (E2E) tests to mimic user journeys. An example flow would be Jest for snapshots and isolated functions, React Native Testing Library for interactive component checks, and Detox (or similar) for real‑device flows. You cover everything from tiny edge cases to broad integration scenarios. This strategy is reliable across the major mobile platforms.
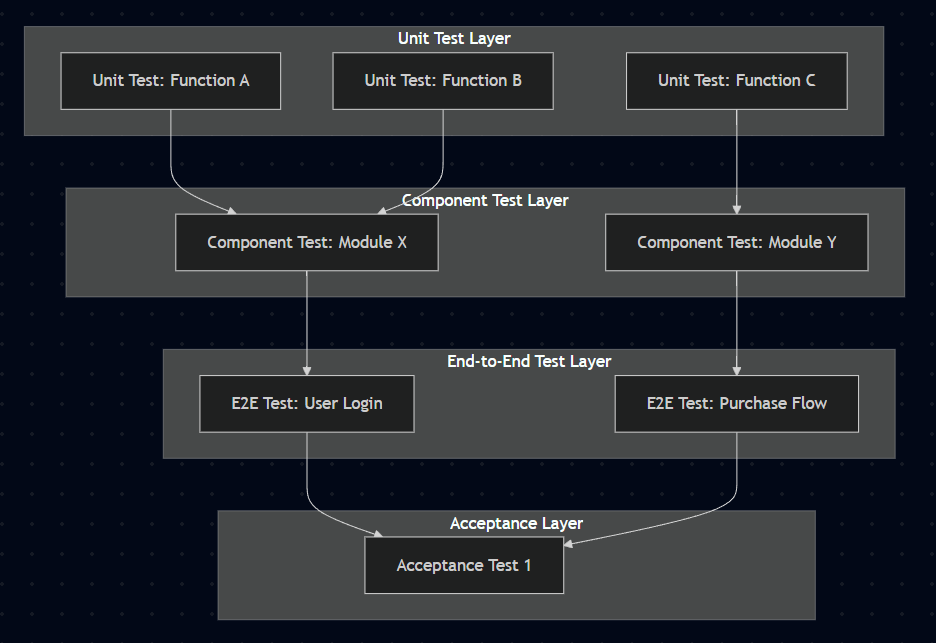
Introduction
Building a mobile app with React Native means writing one codebase that targets both iOS and Android platforms. That convenience brings testing complexity. You must confirm that your UI renders and responds to touch as expected, and that native integrations (camera, geolocation, storage) work seamlessly on devices. Manual QA is important but alone is slow, error-prone, and not scalable. Automated testing catches regressions early, enforces design consistency, and speeds up development.
Challenge
Several factors make React Native testing uniquely challenging:
- Platform differences. iOS and Android often handle gestures, animations, and native modules in slightly different ways. A test that passes on Android may fail on iOS simulators, or vice versa, if you haven’t accounted for subtle UI differences or native behavior.
- Mocking native modules. Modules like AsyncStorage, camera, or push notifications rely on native implementations that aren’t present in pure JavaScript environments. Naively mocking these can lead to false positives and other problems if the mock doesn’t reflect real‑world behavior.
- Snapshot brittleness. Snapshot tests capture the rendered output of a component tree. While powerful for detecting unintended UI changes, they can break whenever you adjust styling, add wrappers, or refactor markup, regardless of whether functionality remains intact.
- Flaky E2E tests. End‑to‑end suites run on emulators or devices and can be sensitive to timing (animations, network delays) or environment issues (CI resource constraints), leading to intermittent failures that are difficult to reproduce.
Solution
1. Unit and Snapshot Testing with Jest
- Setup: Install Jest via your React Native template or Expo’s jest-expo. Configure the Jest preset to handle React Native’s syntax and asset loading.
- Unit tests: Write tests for pure functions, reducers, and utility modules. Keep these fast by mocking any external dependencies.
- Snapshot tests: Use Jest’s snapshot feature judiciously: snapshot critical UI components (buttons, headers, lists) to detect layout regressions. Store snapshots alongside your test files and review diffs in pull requests.
- Mocking: Leverage Jest’s mocking capabilities for native modules. Create manual mocks under a __mocks__ directory for AsyncStorage, NetInfo, or other key dependencies. This ensures consistent behavior across test runs.
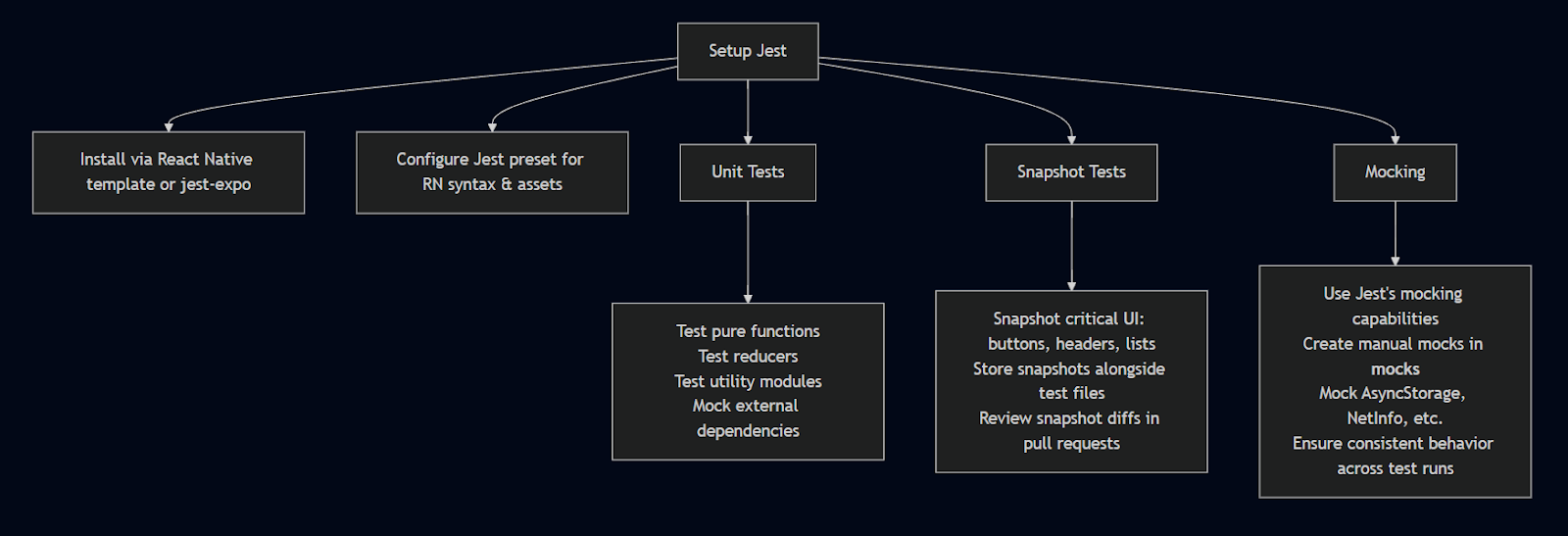
2. Component Testing with React Native Testing Library
- Rendering. Use render() to mount components in a lightweight DOM-like environment.
- Queries. Employ accessibility queries like getByText, getByPlaceholderText, and getByTestId to locate elements in a way that mimics user interaction.
- User events. Simulate presses, text input, scrolls, and other gestures with fireEvent. Verify side effects such as state updates, navigation calls, or API requests by asserting on DOM changes or mock function invocations.
- Avoid implementation details. Test the “what” (behavior), not the “how” (internal state). This makes your tests more resilient to refactors that don’t change user‑visible output.
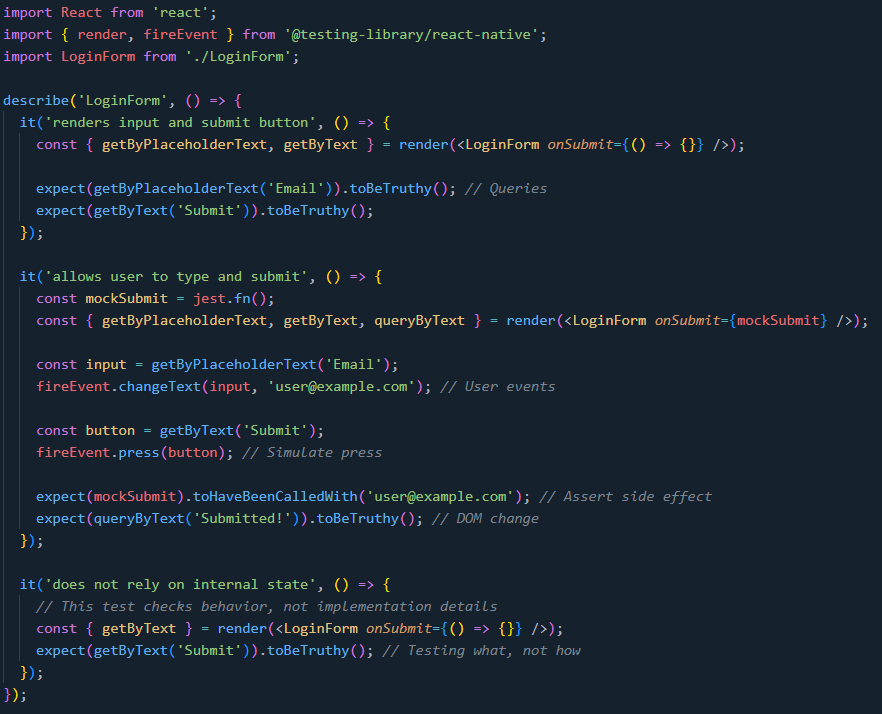
3. End‑to‑End Testing with Detox
- Project configuration. Install Detox CLI and configure your Xcode and Android project settings for a release‑mode build optimized for testing.
- Writing tests. Use Detox’s API to script full user flows such as onboarding, login, or form submissions.For example, a simple login test could look like:
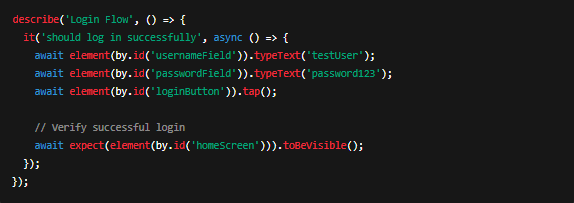
- Running tests. Execute E2E suites on emulators or real devices locally, then integrate them into your CI pipeline. Configure robust retry logic for network‑dependent steps.
- Stabilization strategies. Insert explicit waits for animations or network responses, use Detox synchronization features, and isolate tests into small, independent files to reduce inter‑test interference.
Caveats
- Reset state between tests. Clear AsyncStorage, mocks, and navigation history in setup/teardown hooks to eliminate cross‑test pollution.
- Limit snapshot scope. Only snapshot small, stable components rather than entire screens. This reduces false positives and review overhead.
- Balance speed and coverage. Fast unit tests should cover most logic, component tests handle UI, and E2E tests validate critical flows.
- CI integration. Automate your full test suite on each pull request. Early feedback prevents regressions.
Conclusion
Adopting a layered testing strategy transforms how you build React Native apps. This blend of speed and realism improves code quality and accelerates development by enabling confident refactoring and faster releases. Start by writing a few unit tests today, and gradually layer in component and E2E coverage as your app evolves, expand your coverage over iterations, and weave testing into your daily workflow. The earlier you implement automated testing, the more you’ll ensure long-term code quality and faster iteration on features.
Frequently Asked Questions
What role does Jest play in React Native testing?
Jest is the primary JavaScript testing framework included in React Native templates out of the box, providing tools for unit, snapshot, and mock testing. It allows running fast, isolated tests of functions, reducers, and utilities.
What is the React Native Testing Library (RNTL), and why use it?
RNTL is a companion to Jest that focuses on testing components by rendering them and querying based on accessibility labels or testIDs, mimicking how users interact with the UI. It encourages testing behavior over implementation by providing user-centric queries.
How do I mock native modules in React Native tests?
Native modules such as AsyncStorage or NetInfo lack pure JS implementations, so you create manual mocks in a __mocks__ directory or use Jest’s automocking to stub their behavior.
How do I choose between unit, component, and E2E tests?
Unit tests should cover pure functions and business logic for speed; component tests verify UI behavior and interactions; and E2E tests confirm full user journeys for real-world confidence. Balancing the three layers avoids overloading CI.
What are the best practices for organizing React Native test files?
Place test files alongside source files using a .test.js extension for clarity; group them by feature or component for maintainability; and share common setup and teardown logic in Jest’s configuration or setup files to DRY up configurations.
.jpeg)
Modurotolu Olokode
Modurotolu Olokode is a seasoned full-stack engineer with a decade of experience in building scalable applications. Modurotolu is passionate about solving problems with technology and loves sharing insights that empower developers to make informed technical decisions.
Article by Gigson Expert